React File Picker
The File Picker is an open-source, plug-and-play, React component that connects to many cloud storage APIs and offers easy file uploads and downloads between your app and any cloud storage service. This NPM package works with the File Storage API and allows your users to select, search and upload files to multiple services.
This guide will get you up and running with the File Picker component. To get started, follow these 3 steps:
Step 1: Setup Apideck
Create an account
If you haven't already, head over to our Signup page and create an account. Choose an application name and a subdomain. Afterward, you will be redirected to the Apideck dashboard.
Enable Unified APIs and connectors
Go to the Unified APIs page in the Apideck dashboard. Choose one or more Unified APIs to enable. You'll see a list of the available connectors for each Unified API. Choose a couple of connectors to enable. The Unified APIs and connectors you select become available to your users in Vault.
Get your API key and Application ID
Go to the API Keys page in the Apideck dashboard. Copy your application ID and API key. If your API key ever gets compromised, you can regenerate it on this page.
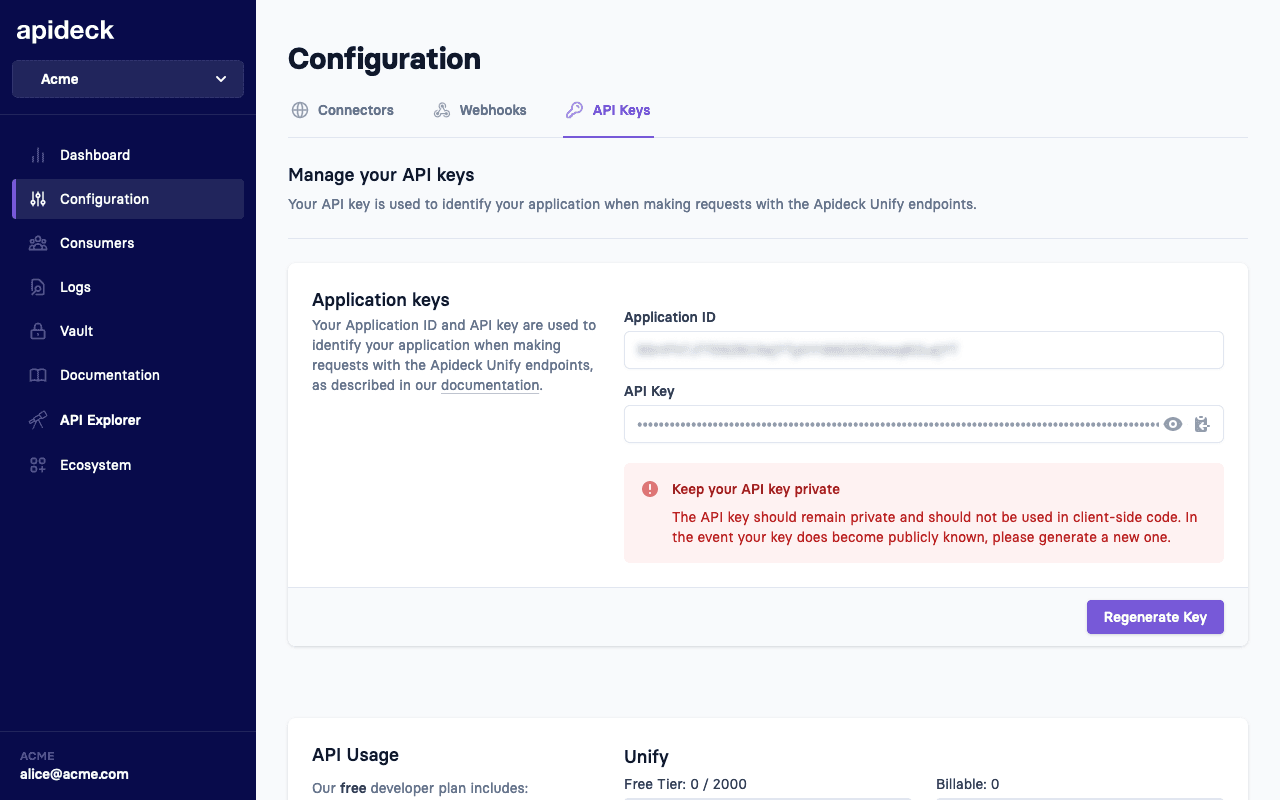
Step 2: Create a session
Vault lets your users (called consumers in Apideck) easily connect and configure integrations. You can create a consumer through a Vault session through the following endpoint https://developers.apideck.com/apis/vault/reference#tag/Sessions.
Most of the time, this is an ID of your internal data model that represents a user or account in your system. E.g., account:12345. If the consumer doesn't exist yet, Vault will upsert a consumer based on your ID.
Note 🚨
Session creation should always happen server-side to prevent token leakage.
Use the API call below to create a session for a consumer. This will return a Vault URL that you forward to a consumer to connect integrations.
You can also use our Node SDK to create a session.
Below is an example of creating a Serverless Function to create a session inside a Next.js project:
The returned
dataobject will include the
session_tokenthat you can use pass as the
tokenprop to the
FilePickercomponent.
Step 3: Add the File Picker
Install the NPM package.
Next, pass the JSON Web Token returned from the Create Session call together with your Application ID and a consumer ID to the FilePicker component.
The component is styled using Tailwind CSS. If you were to use the Vault component in a project that also uses Tailwind CSS, you can omit the CSS file import, and include the package path in the config file. Doing this will tell Tailwind to scan the package and include the needed styles in your bundle and you won't have duplicates in your CSS.
You can also provide a file through the
fileToSaveprop that will force the FilePicker to go into "Upload" mode. This will allow the user to select the connector and folder that the file needs to get saved to.
Demo
The code block below shows a quick demo on how you could use the FilePicker component. Click the button to execute the code that's shown below. In the demo we use the
@apideck/file-picker-jspackage, which can be used in any JavaScript application. Loading
Properties
Property | Type | Required | Default | Description |
---|---|---|---|---|
token | string | true | - | The JSON Web Token returned from the Create Session call |
open | boolean | false | false | Opens the file picker if set to true |
onSelect | event | true | - | The function that gets called when a file is selected |
onClose | event | false | Function that gets called when the modal is closed | |
trigger | element | false | - | The element or component that should trigger the File Picker modal on click |
title | string | false | Apideck File Picker | Title shown in the modal |
subTitle | string | false | Select a file | Subtitle shown in the modal |
showAttribution | boolean | false | true | Show "Powered by Apideck" in the backdrop of the modal backdrop |