How to get the raw response from downstream APIs
Apideck's Unified API provides realtime access to data from underlying APIs, offering a powerful feature called "Raw Mode" across all its Unified APIs. This feature allows you to retrieve the unprocessed response directly from the integrated connector, alongside Apideck's normalized data. Raw Mode gives you the best of both worlds: access to raw data from the original API and the standardized data processed by Apideck.
Raw Mode is particularly useful for:
- Debugging: Examine the original API response for troubleshooting.
- Accessing additional data: Retrieve information not included in the normalized response.
- Maintaining full context: Get the complete output from the underlying API when needed.
This guide will walk you through using Raw Mode, explore its benefits, and provide best practices for implementation.
Note
If you're on a Growth plan or higher we recommend using the custom field mappings feature to map the raw data to the data model you need.
What is Raw Mode?
Raw mode returns the original, unmodified response from the service you're integrating with, bypassing Apideck's normalization process. This can be invaluable for debugging, accessing additional data not included in the normalized response, or when you need the full context of the API's output.
Using raw mode may increase the response size and potentially introduce extra latency, so it's recommended to use it primarily for debugging rather than in production environments.
How to Enable Raw Mode
When you include
raw=truein your request, Apideck will return the raw response from the underlying API. This can be useful for debugging purposes, as it allows you to see the full, unprocessed response from the service you're integrating with. For example:
https://unify.apideck.com/crm/leads?raw=true
The request to the Unified API will have a
_rawproperty in the response body that contains the raw response from the underlying API. If multiple pages of data are returned, the
_rawproperty will contain an array of pages with the raw response for each page.
{ "status_code": 200, "status": "OK", "service": "hubspot", "resource": "leads", "operation": "all", "data": [ { "id": "551", "name": "Emilia Earhart", "first_name": "Emilia", "last_name": "Earhart", "updated_at": "2024-04-05T07:51:00.266Z", "created_at": "2020-10-02T14:48:17.728Z" } ], "_raw": { "pages": [ { "results": [ { "id": "551", "properties": { "address": "B221, Bakers Street", "assigned_csm": "Kristof W.", "associatedcompanyid": "4562962674", "city": "Antwerp", "company": "Apideck", "country": "Belgium", "createdate": "2020-10-02T14:48:17.728Z", "custom_field": "", "email": "e.earhart@noemail.com", "firstname": "Emilia", "hs_object_id": "551 }, "createdAt": "2020-10-02T14:48:17.728Z", "updatedAt": "2024-04-05T07:51:00.266Z", "archived": false }, ], "paging": { "next": { "after": "7202", "link": "https://api.hubapi.com/crm/v3/objects/contacts?properties=company%2Cassociatedcompanyid%2Cfirstname%2Clastname%2Cemail%2Cphone%2Cjobtitle%2Cwebsite%2Chubspot_owner_id%2Clifecyclestage%2Ccity%2Ccountry%2Caddress%2Czip%2Cstate%2Cassigned_csm%2Ccustom_field%2Cknown_via%2Ct_shirt_size&limit=20&after=7202" } }, "_headers": {}, "_status": 200, "_namedParameters": { "raw": "true", "properties": "company,associatedcompanyid,firstname,lastname,email,phone,jobtitle,website,hubspot_owner_id,lifecyclestage,city,country,address,zip,state,assigned_csm,custom_field,known_via,t_shirt_size", "limit": 20 } } ] } }
Access the raw response from the logs
You can access the raw response from the logs in the Apideck Dashboard. Next to the request happening to the Unified API, you can see the call to the underlying API via the child requests.
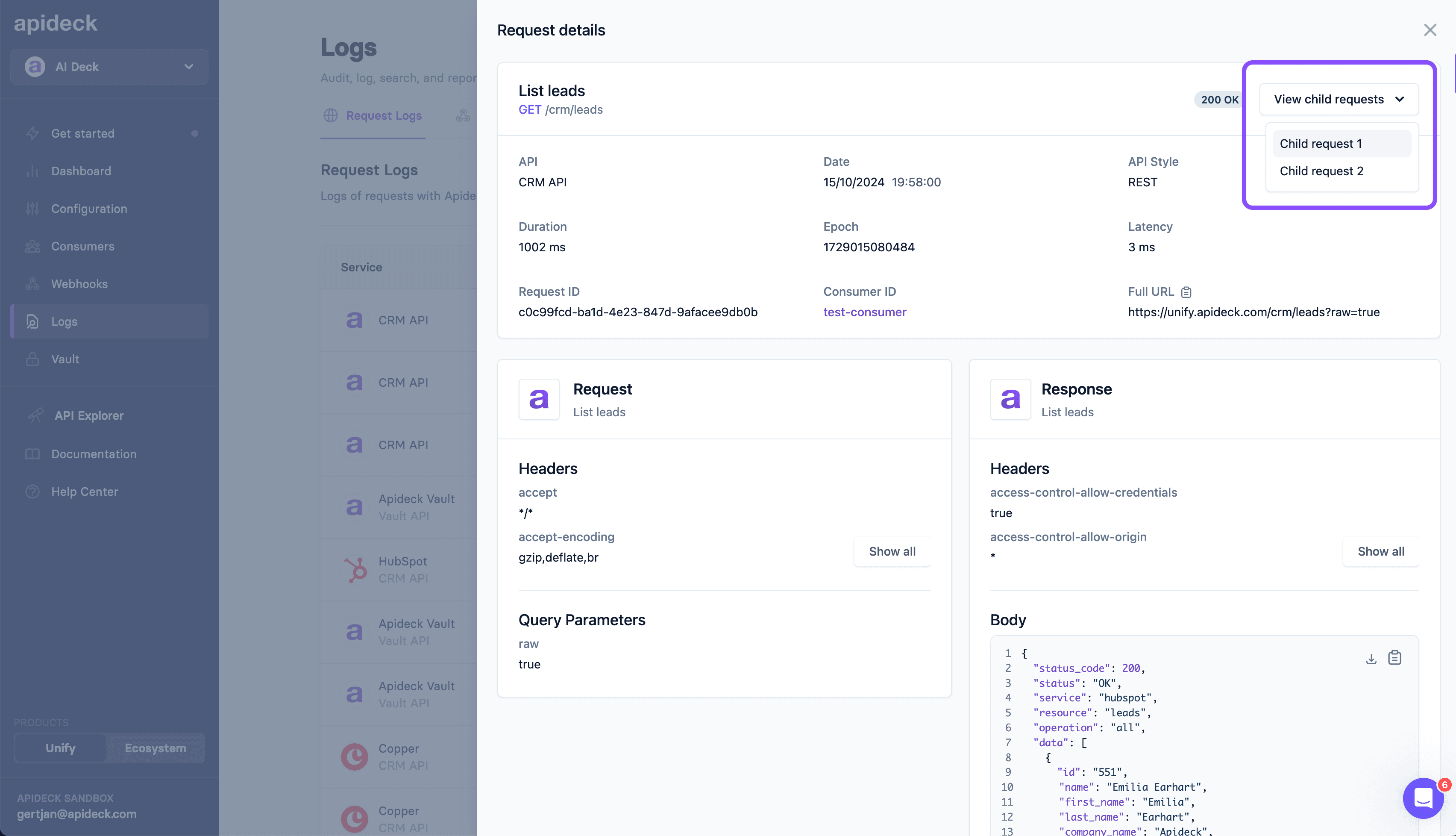
Troubleshooting
Some APIs may have additional requirements or restrictions for getting all the data depending on the scopes and auth method used.
How is this different from the Proxy API
Sometimes you need data that sits outside of the API endpoints offered on our Unified Data Model. You can use the Proxy API to access these endpoints. You can read more about using the Proxy here.